第一章 如何用vue.js来写一个hello world程序
1、目录结构
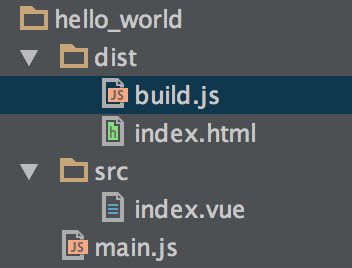
2、index.vue
<style>
h2{
color: red;
}
</style>
<template>
<h2>Hello, World!</h2>
</template>
<script>
module.exports = {
data: function(){
return {};
}
}
</script>
3、入口文件main.js
var Vue = require("vue");
var hello = require("./src/index.vue");
new Vue({
el: "body",
components: {
hello: hello
}
});
4、webpack.config.js
var path = require('path');
var ROOT_PATH = path.resolve(__dirname);
var APP_PATH = path.resolve(ROOT_PATH, 'test/hello_world');
var BUILD_PATH = path.resolve(ROOT_PATH, 'test/hello_world/dist');
module.exports = {
debug: true,
entry: APP_PATH+"/main.js",
output: {
path: BUILD_PATH,
filename: 'build.js'
},
module: {
loaders: [
{
test: /\.vue$/,
loader: 'vue'
},
{
test: /\.(png|jpg|eot|svg|ttf|woff)/,
loader: 'url?limit=40000'
}
]
}
}
5、在命令行执行webpack命令,生成build.js
webpack
6、index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>hello world</title>
</head>
<body>
<hello></hello>
<script src="build.js"></script>
</body>
</html>